The Script Editor is a simple and functional utility for writing and executing VB or C# script against the currently opened ABACUS file (Libraries are not supported in scripting). It has the usual File Open, Close and Save commands, so you can save and retrieve scripts you write. It also has a basic run, compile and debug facility. The Script Manager allows you to easily execute the various scripts that you have written by placing a menu-item for them directly in the Tools menu.
To access the Script Editor
1.Ensure you have the ABACUS file, containing your populated architecture, open.
2.Select Tools | Script Editor and the Script Editor window will open. Then either;
3.Open an existing script via File | Open in the Script Editor menus,
- or -
3.Open an Example script via the Example item in the Script Editor menus,
- or -
3.Set the default Language to either VB or C# via the Language item in the Script Editor menus,
- or -
3.Open scripting specific Help via the Help item in the Script Editor menus
When writing scripts, you must adhere to either the VB or C# syntax as per the setting under the Language item in the Script Editor menus.
NOTE:
•To use standard VB functions like MsgBox() you must include the Imports Microsoft.VisualBasic statement at the beginning of your script. This was not necessary before Version 3.0 of ABACUS.
•This help does not include information on how to write VB script, nor does it contain a VB reference. Feel free to write your scripts in your favourite IDE or editor, then copy and paste them into the Script Editor.
To write a useful script you will need to reference the ABACUS ObjectModel. It is made available to your script by including the Imports ABACUS.ObjectModel statement at the head of your script. A complete reference to the entire ABACUS object model is included with the Script Editor. You can find it by selecting Help | Contents from the Script Editor window.
The script editor includes many useful features for writing scripts, including AutoComplete as shown in the screenshot below. However, for an even richer user experience MS Visual Studio can be integrated with ABACUS and detailed instructions are available here.
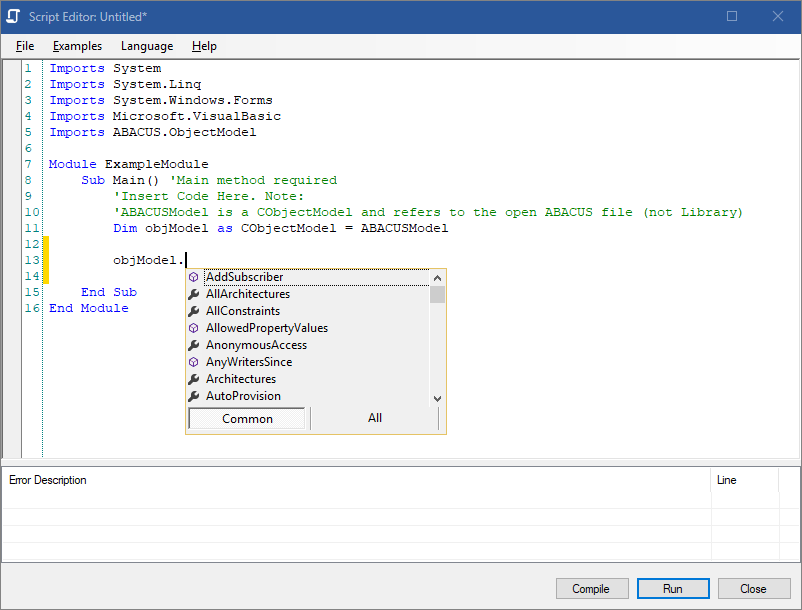
Script Editor with AutoComplete
Personal Scripts
To use the Personal Script Manager
1.Ensure you have the ABACUS file, containing your populated architecture, open.
2.Select Tools|Personal Scripts|Manage Personal Scripts.
3.Click on the Add icon and an Open window appears.
4.Browse to the ABACUS Script (.abacusscript, .vb or .cs) file you want to add to the Tools menu.
5.Click OK and a reference to the script will be shown in the Script Manager window. Enter the name for the script as you wish it to appear in the Tools menu. Note: The Script Manager window can be resized to see the full path if necessary.
6.Repeat steps 3-5 to create links to more ABACUS scripts.
7.Click Close to close the Script Manager and note that the ABACUS script(s) now appear under the Tools menu.
Using Scripts
To run a personal script
Either;
•Open, type in, or copy and paste in the script you want to run, so it is displayed in the main window of the Script Editor, then click the Run button.
- or -
•Select Tools and then the ABACUS Script name menu item as created with the Manage Project Scripts dialogue.
- or -
•Select Tools|Personal Scripts and then the ABACUS Script name menu item as created with the Manage Personal Scripts dialogue.
- or -
•Open the script via Open with.. -> ABACUS which will open ABACUS and the script will run automatically (if ABACUS is set to the default app for the script, you can simply double-click the script for this).
Note
If your script contains errors, the Error Description section at the bottom of the Script Editor will list the details including the line numbers.
Tip
Each individual error in the Error Description section can be double-clicked to take you to the corresponding line in the script.
Scripting Compilation Errors
Compilation errors occur when the syntax of the script code is not correct. These errors will show up at the bottom of the window in the Error section, with a description of the error, and a line number. It is the line number which distinguishes a compilation error from a Runtime error (see below), which will not have a line number. Compilation errors can be double clicked to jump to and highlight the specific line in question.
Scripting Runtime Errors (Important)
Runtime errors occur after the script code has been successfully compiled without errors, but an error occurs at runtime (see examples down further). Runtime errors are important as they can result in corrupted models, when objects created and referenced by other objects in the model, are not themselves part of the model (usually because a runtime error has occurred before the code that would have placed them in the model somewhere, was executed).
If your script creates objects to be added to the model, you can avoid corruption caused by a runtime error by saving your script, closing scripting, and performing an undo of the changes that were created by the script (if script execution did not complete normally). However, there are also other ways to avoid any corruption occurring (see below). Note: Any scripts that merely read from the model and do not write to it, are safe and will not cause any problems, regardless of whether runtime errors occur during their execution.
To avoid corruption, when writing script code that is creating objects and adding them to the object model, it is suggested that the objects are added to the model as soon as possible if they are referenced by other objects.
For example, if you are creating a new connection, and 2 new components, and then attaching them together, it is best to create the first component, add it to the model, then the second and add it, and then create the connection and add it, and then finally create the attachments. This means that if there were to be a runtime error that interrupts the script execution, all objects that are required to be in the objectmodel before an attachment is created, are in the model. Corruption may occur if, for example, only the connection had been added, the attachments made, and then a runtime error occurred before the script finally added the new components to the model.Corruption can occur whenever an object that is referenced by another object in the objectmodel, is itself not in the objectmodel.
Example runtime errors include:
•Object reference not set to an instance of an object - this occurs when an object variable is a null reference, and a method or property on that object is being used. Null references can be checked by 'If objectVariable Is Nothing Then...'
•Index was outside the bounds of the array - this occurs when an array is being accessed with an index that is outside the bounds - e.g. less than 0 or equal to or greater than the Length/Count of the array. Array or Collection length can be checked by the .Length or .Count properties respectively.
•No Permission Exception - You do not have permission to... - this one occurs if you do not have permission to make the given change
•Primary Key Exception - Duplicate/Missing Name/ID - this one will occur if a change is made to a unique attribute that conflicts with another object. E.g. Architectures must be unique by name, likewise with Standards.
•Referential Integrity Exception - Setting a reference field to an object which is not valid. E.g. adding 'nothing' or null as a child to many of the collections in the objectmodel.
•Zero Length Exception - this may occur if you try to set the name of an object to an empty string. Most objects require their names to have at least 1 character.
Imports statements
Note that at the start of the skeleton scripts there are statements such as Imports ABACUS.ObjectModel. These statements will not include a dll that is not already referenced by the script - their purpose is to provide a 'shortcut' to having to specify the entire name of a class.
For example, to declare a variable of type CComponent (representing a component in ABACUS), with the Imports ABACUS.ObjectModel statement towards the top of the script, would only require it to be declared as dim comp as CComponent, because the compiler knows to look in the ABACUS.ObjectModel namespace for the CComponent class. Without the imports statement, it would have to be declared as dim comp as ABACUS.ObjectModel.CComponent. An assembly (such as mscorlib.dll, or System.Windows.Forms.dll) can contain more than one namespace - the Imports statement is for these namespaces, not for the assemblies themselves. The ABACUS.ObjectModel.dll assembly only has the single ABACUS.ObjectModel namespace accessible via Scripting.
Automatically referenced assemblies
The following DLLs are referenced automatically by Scripting:
•mscorlib.dll
•System.dll
•System.Drawing.dll
•System.Windows.Forms.dll
•Microsoft.VisualBasic.dll (implicitly referenced by the compiler)
•ABACUS.ObjectModel.dll
To make additional references to either other .NET assemblies, or your own assemblies, see the following section on the #ImportDLL statement.
#ImportDLL Statement
Starting in ABACUS 3.1, you can reference additional assemblies that you may require for scripting with the use of the #ImportDLL statement. For example, if you wanted to access a custom DLL of yours and utilise the functionality in it, you would place it in the ABACUS directory and include the statement as the first line(s) in your script. If it is a .NET dll, then normally you do not need to enter the entire path, just the DLL.
•#ImportDLL C:\Program Files\Avolution\ABACUS\YourDLL.dll
•#ImportDLL System.XML.dll
Some other things to note:
1.Because the DLLs need to be found at compile and runtime, this restricts custom (non .NET) DLLs to being placed in the ABACUS program folder only.
2.#ImportDLL statements must be the first line(s) of a script - if you have 5 statements, then they should be on the first 5 lines of the script
3.An #ImportDLL statement placed anywhere but the first lines of the script (e.g. if it was placed after a comment or an Imports statement) will not work correctly and/or may cause unrelated compiler errors.
#ImportDLL with Office Assemblies
If you have Microsoft Office installed, and your script requires the Office Interop assemblies, it is possible to reference them simply by using #ImportDLL with the assembly name (as listed below, e.g. #ImportDLL Excel). Using this method has the advantage that an absolute dll path does not have to be used, so the script is more portable (should work on both Office 2003 and 2007 without editing the script).
When you use this feature, the GAC (Global Assembly Cache) is searched for the interop assembly, and the script compiles with the path to that assembly if found. If it's not found, scripting will check the ABACUS program directory (which contains the Excel and Visio interops only). If the requested interop is not found by this time, a warning is shown.
The interops are typically not installed in the GAC if you have installed Microsoft Office before installing the .NET Framework (which is part of the full ABACUS installation). You can install them by performing the Change option for Microsoft Office from Add/Remove Programs, and choose .NET Programmability options. For more info, see this article on MSDN.
The assemblies for which this shortcut is supported are:
•Access
•Access.Dao
•Excel
•Graph
•MSProject
•Outlook
•OutlookViewCtl
•Owc11
•PowerPoint
•Publisher
•SmartTag
•Visio
•Visio.SaveAsWeb
•VisOcx
•Word
It is not entirely clear if all the above assemblies are useful, but they have been included in this feature regardless as some users may have a need for even the obscure assemblies.

See Also
Simulating your architecture
© 2001-2024 Avolution Pty Ltd, related entities and/or licensors. All rights reserved.
|